What is Exception Handling in Java? How to use Exception Handling in Java – Java Tutorial
Problem Definition:
Give the basic form of an exception handling block.
OR
Define the role of Exception Handling in software development.
OR
Define an exception in Java. Explain the exception handling mechanism with an example.
OR
Explain exception handling with a suitable code.
Solution:
The exception is an unusual situation in a program that may lead to a crash the program. Usually, it indicates the error.
Video Tutorial – Exception Handling in Java
Various keywords used in handling the exception are –
try – A block of source code that is to be monitored for the exception.
catch – The catch block handles the specific type of exception along with the try block. Note that for each corresponding try block there exists the catch block.
finally – It specifies the code that must be executed even though an exception may or may not occur.
throw – This keyword is used to throw a specific exception from the program code.
throws – It specifies the exceptions that can be thrown by a particular method.
The Java code that you may think may produce an exception is placed within the try block and the exception should be handled in the associated catch block.
Let us see one simple program in which the use of try and catch is done in order to handle the exception divide by zero.
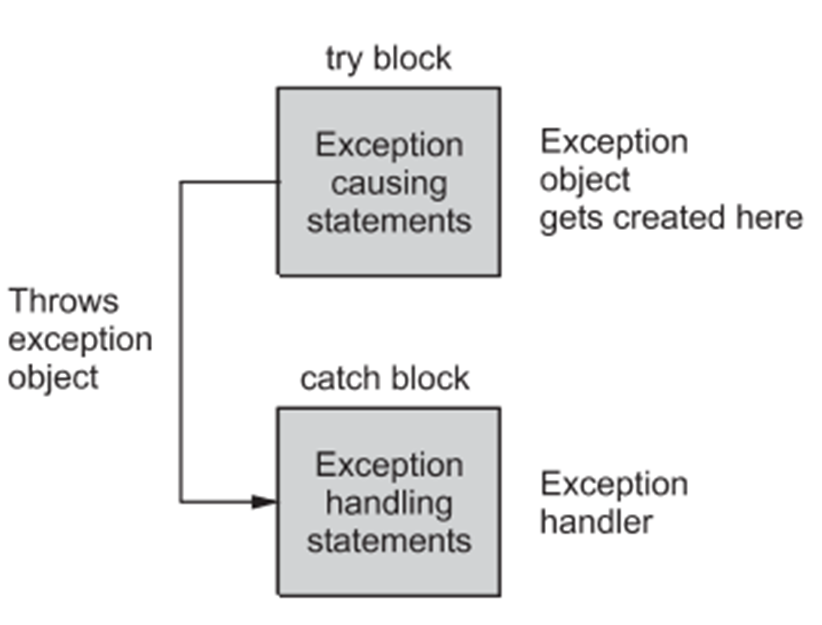
Exception Handling – Try-catch Block – Example
class RunErrDemo { public static void main(String[] args) { int a = 10, b = 0, c; try { c = a/b; System.out.println(c); } catch(ArithmeticException e) { System.out.println("\n Divide by zero"+e); } } }
Output:
Divide by zero: javalang.ArithmeticException: / by zero
The value of a: 10
The value of b: 0
Note that even if the exception occurs at some point, the program does not stop at that point.
Multiple Catch blocks in Java
It is not possible for the try block to throw a single exception always.
There may be situations in which different exceptions may get raised by a single try block statement and depending upon the type of exception thrown it must be caught.
To handle such a situation multiple catch blocks may exist for the single try block statements.
The syntax for the single try and multiple catch is –
try
{
…//exception occurs
}
catch(Exception_type1 e1)
{
…//exception is handled here
}
catch(Exception_type2 e2)
{
…//exception is handled here
}
catch(Exception_typen en)
{
…//exception is handled here
}
Multiple Catch Example Program
class MultipleCatchDemo { public static void main (String args []) { int a[] = new int [3]; try { for (int i = 1; i <3; i++) { a[-1] = i *i; // ArrayIndexOutOfBoundsException due to negative index } for (int i = 0; i <3; i++) { a[i] = i/i; //ArithmeticException due to divide by Zero } } catch (ArrayIndexOutOfBoundsException e) { System.out.printin ("The array index is out of bounds"); } catch (ArithmeticException e) { System.out.println ("Divide by zero error"); } } }
Output:
The array index is out of bounds
Note: If we comment the first for loop in the try block and then execute the above code we will get the following output-
Divide by zero error
Finally Block in Java Exception Handling
Sometimes because of the execution of the try block, the execution gets abruptly stops.
And due to this some important code (which comes after throwing off an exception) may not get executed.
That means, sometimes try block may bring some unwanted things to happen.
The finally block provides the assurance of execution of some important code that must be executed after the try block.
Even though there is any exception in the try block the statements assured by finally block are sure to execute.
These statements are sometimes called clean-up code.
The syntax of finally block is
finally
{
//clean up code that has to be executed finally
}
The finally block always executes. The finally block is to free the resources.
class finallyDemo { public static void main(String args[]) { int a=10, b=0, c; try { c=a/b; } catch(ArithmeticException e) { System.out.println("In catch block: "+e); } finally { System.out.println(“Program Finished"); } } }
Output
In catch block: javalang.ArithmeticException: / by zero
Program Finished
Throws Keyword in Exception Handling Java
throws is a keyword in Java that is used in the signature of a method to indicate that this method might throw one of the listed type exceptions.
The caller to these methods has to handle the exception using a try-catch block.
When a method wants to throw an exception then keyword throws is used.
method name(parameter list) throws exception list
{
}
Let us understand this exception handling mechanism with the help of a simple Java program.
class ExceptionThrows { static void fun(int a, int b) throws ArithmeticException { int c; c=a/b; System.out.println(c); } public static void main(String args[]) { try { fun(10,5); } catch(ArithmeticException e) { System.out.println("Caught exception: "+e); } } }
Output
Caught exception: javalang.ArithmeticException: / by zero
Program Explanation:
In the above program, the method fun is handling the exception divide-by-zero.
This is an arithmetic exception hence we write
static void fun(int a, int b) throws ArithmeticException
This method should be of static type.
Also note calling method is responsible for handling the exception the try-catch block should be within the fun.
Throw Keyword in Exception Handling Java
For explicitly throwing the exception, the keyword throw is used.
The keyword throw is normally used within a method.
We can not throw multiple exceptions using throw.
class ExceptionThrow { static void fun(int a, int b) { int c; if(b==0) throw new ArithmeticException("Divide By Zero!!!"); else System.out.println(a/b); } public static void main(String args[]) { try { fun(10,0); } catch(ArithmeticException e) { System.out.println("Caught exception: "+e); } } }
Output:
Caught exception:
java.lang.ArithmeticException: Divide By Zero!!!
Difference between throw and throws
Throw | Throws |
For explicitly throwing the exception, the keyword throw is used. | For declaring the exception, the keyword throws is used. |
The throw is followed by an instance. | Throws is followed by the exception class. |
The throw is used within the method. | Throws is used with method signature. |
We cannot throw multiple exceptions. | It is possible to declare multiple exceptions using throws. |
Benefits of Exception Handling
Following are the benefits of exception handling –
1. Using exceptions, the main application logic can be separated out from the code which may cause some unusual conditions.
2. When the calling method encounters some error then the exception can be thrown. This avoids crashing of the entire application abruptly.
3. The working code and the error handling code can be separated out due to the exception handling mechanism. Using exception handling, various types of errors in the source code can be grouped together.
Summary:
In this article, we understood, What is Exception Handling in Java? How to use Exception Handling in Java – Java Tutorial. If you like the tutorial share it with your friends. Like the Facebook page for regular updates and the YouTube channel for video tutorials.