Control Statements in Java – Java Tutorial
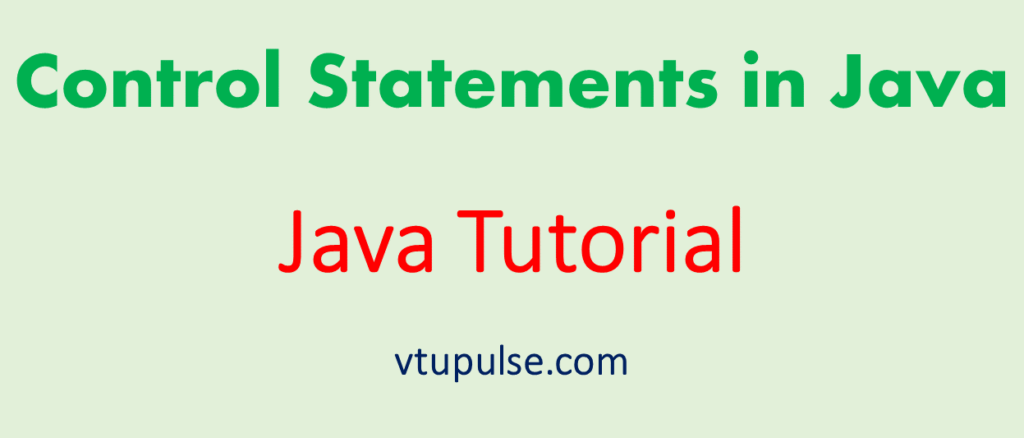
Problem Statement
List and Explain different Control Statements in Java.
Solution:
Programmers can take decisions in their program with the help of control statements. Various control statements that can be used in Java are,
1. if statement
2. if else statement
3. while statement
4. do… while statement
5. switch case statement
6. for loop
Let us discuss each of the control statements in detail.
1. if statement
The if statement is of two types
Simple if statement: The if statement in which only one statement is followed by that statement.
Syntax
if(apply some condition)
statement
For example
if(a>b)
System.out.println(“a is Bning!”);
Compound if statement: If there is more than one statement that can be executed when if condition is true. Then it is called the compound if statement. All these executable statements are placed in curly brackets.
Syntax
if(apply some condition)
{
statement 1
statement 2
statement n
}
2. if…else statement
The syntax for if…else statement will be –
if(condition)
statement
else
statement
For example
if(a>b)
System.out.println(“a is big”)
else
System.out.println(“b :big brother”)
The if…else statement can be of compound type even.
For example
If(raining= =true)
{
System.out.println(“I won’t go out”);
System.out.println(“I will watch T.V. Serial”);}
else
{
System.out.println(“I will go out”);
System.out.println(“And will meet my friend”);
}
if…else if statement
The syntax of if…else if statement is
if(is condition true?)
statement
else if(another condition)
statement
else if(another condition)
statement
else
statement
Example Program
class ifelsedemo { public static void main(String[] args) { int x=111, y=120, z=30; if(x>y) { if(x> z) System.out.println("The x is greatest"); else System.out.println("The z is greatest"); } else { if(y> z) System.out.printin("The y is greatest"); else System.out.println("The z is greatest"); } } }
3. while statement
This is another form of while statement which is used to have an iteration of the statement for any number of times.
The syntax is
while(condition)
{
statement 1;
statement 2;
statement 3;
• • •
statement n;
}
class whiledemo { public static void main(String args[]) { int count=1,i=0; while(count< =5) { i=i+1; System.out.println("The value of i= "+i); count+ +; } } }
Output
The value of i= 1
The value of i= 2
The value of i= 3
The value of i= 4
The value of i= 5
4. do… while statement
This is similar to the while statement but the only difference between the two is that in the case of do…while statement the statements inside the do…while must be executed at least once.
This means that the statement inside the do…while the body gets executed first and then the while condition is checked for the next execution of the statement, whereas in the while statement first of all the condition given in the while is checked first and then the statements inside the while body get executed when the condition is true.
Syntax
do {
statement 1;
statement 2;
statement 3;
• • •
statement n;
} while(condition);
class dowhiledemo { public static void main(String args[]) { int count=1,i=0; do { i=i+1; System.out.println("The value of i= "+i); count++; }while(count< = 5); } }
Output
The value of i= 1
The value of i= 2
The value of i= 3
The value of i= 4
The value of i= 5
5. switch statement
You can compare the switch case statement with a Menu-Card in the hotel.
You have to select the menu then only the order will be served to you. Here is a sample program that makes use of a switch case statement –
class switchcasedemo { public static void main(String args[]) throws java.io.IOException { char choice; System.out.printIn(" \tProgram for switch case demo'); System.out.println("Main Menu"); System.out.println("1. A"); System.out.println("2. B'); System.out.println("3. C'); System.out.println("4. None of the above"); System.out.println("Enter your choice'): Choice = (char)System.in.read(); switch(choice) { case '1':System.out.println('You have selected A'); break; case '2':System.out.println('You have selected B'); break; case '3':System.out.println('You have selected C'); break; default:System.out.println("None of the above choices made'); } } }
Output
Program for switch case demo
Main Menu
1. A
2. B
3. C
4. None of the above
Enter your choice 2
You have selected B
6. For loop
for is a keyword used to apply loops in the program.
Like other control statements for loop can be categorized in simple for loop and compound for loop.
Simple for loop :
for (statement 1; statement 2; statement 3)
execute this statement;
Compound for loop :
for(statement 1; statement 2; statement 3)
{
execute this statement;
execute this statement;
execute this statement;
}
Here Statement 1 is always for initialization of conditional variables, Statement 2 is always for terminating condition of the for loop, and Statement 3 is for representing the stepping for the next condition.
class forloop { public static void main(String args[]) { for(int i=0;i< =5;i+ +) System.out.println("The value of i: "+i); } }
Output
The value of i: 0
The value of i: 1
The value of i: 2
The value of i: 3
The value of i: 4
The value of i: 5
Summary:
In this article, we understood How to use Control Statements in Java – Java Tutorial. If you like the tutorial share it with your friends. Like the Facebook page for regular updates and the YouTube channel for video tutorials.