Arrays in Java – Java Tutorial
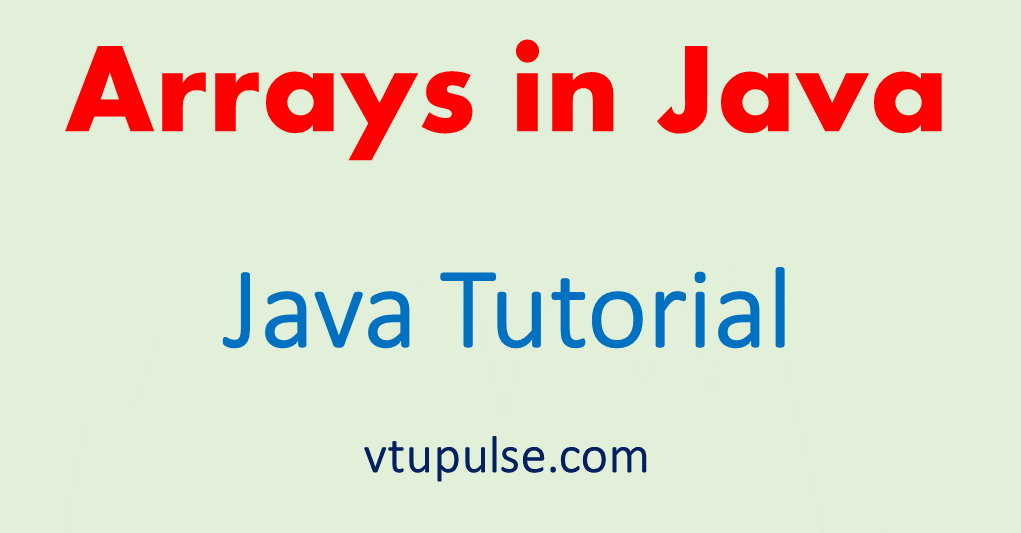
Problem Statement
How arrays are defined in Java? Explain with a programming example.
Solution:
There are three types of arrays in Java Programming Language.
- Single Dimensional Arrays
- Two Dimensional Arrays
- Ragged Arrays
1. Single Dimensional Array in Java
The array is a collection of similar types of elements. Thus grouping of similar types of elements is possible using arrays. Typically arrays are written along with their size of them.
The syntax of declaring an array is –
data_type array_name[ ];
and to allocate the memory –
array_name=new data_type[size];
where array_name represents the name of the array, new is a keyword used to allocate the memory for arrays, data_type specifies the data type of array elements and size represents the size of an array. based on arrays.
For example :
a=new int[10];
After this declaration the array a will be created as follows Array a[10]

That means after the above-mentioned declaration of the array, all the elements of the array will get initialized to zero.
Note that, always, while declaring the array in Java, we make use of the keyword new, and thus we actually make use of dynamic memory allocation. Therefore arrays are allocated dynamically in Java.
Single Dimensional Array in Java – Program
class SampleArray { public static void main(String[] args) { int a[]; a=new int[5]; System.out.println("Storing the numbers in array"); a[0]=1; a[1]=2; a[2]=3; a[3]=4; a[4]=5; System.out.println("The element at a[2] is: " +a[2]); System.out.println("The element at a[4] is: " +a[4]); } }
Output
Storing the numbers in array
The element at a[2] is : 3
The element at a[4] is : 5
Another way of initialization of array is
int a[ ]= {1,2,3,4,5};
Declare and Initialize Array Elements
That means, as many numbers of elements are present in the curly brackets, that will be the size of an array.
In other words, there is a total of 5 elements in the array and hence the size of the array will be 5.
class SampleArray { public static void main(String args[]) { int a[] = {1, 2, 3, 4, 5}; System.out.println("Storing the numbers in array"); System.out.println("The element at a[2] is: " +a[2]); System.out.println("The element at a[4] is: " +a[4]); } }
Output
Storing the numbers in array
The element at a[2] is : 3
The element at a[4] is : 5
Write a program that creates and initializes a four integer element array. Calculate and display the average of its values.
class AvgDemo { public static void main(String[] args) { int a[]= {10,20,30,40}; int sum=0,avg; for(int i=0;i<4;i++) { sum=sum+ a[i]; } System.out.println("Sum= "+sum); avg=sum/4; System.out.println("\nAverage=" +avg); } }
Output
Sum= 100
Average= 25
2. Two Dimensional Array in Java
The two-dimensional arrays are the arrays in which elements are stored in rows as well as in columns.
For example Rows The two arrays can be declared and initialized as follows Syntax
datatype array_name[][]=new data_type[size][size];
For example:
int a[][]=new int[3][3];
Let us demonstrate a java program that is using two-dimensional arrays.
class Sample2DArray { public static void main(String args[]) { int a[][]=new int[3][3]; int k=0; System.out.println("\tStoring the numbers in array"); for(int i=0;i<3;i++) { for(int j=0;j<3;j++) { a[i][i]=k+10; k=k+10; } } System.out.println("You have stored..."); for(int i=0;i<3;i++) { for(int j=0;j <3;j++) { System.out.print(" "+a[i][j]); } System.out.println(); } } }
OUTPUT:
Storing the numbers in array
You have stored…
10 20 30
40 50 60
70 80 90
3. Ragged Array in Java
In two dimensional array, each row has an equal number of columns. But if you want each row should contain a different number of columns, then we can use a ragged array. In a Ragged array, each dimension can have a different size.
For example {{1,2,3,4}, {5,6}, {7,8,9}} is a ragged two-dimensional array.
In this case, the first row has 4, the second row has 2 and the third row has 3 columns.
Following is a simple Java program that represents the use of the ragged array.
public class RaggedArray { public static void main(String[] args) { int A[] = new int[3][]; A[0] = new int[4]; A[1] = new int[2]; A[2] = new int[3]; System.out.println("Total Number of Rows: " + A.length); // 1st row A[0][0] = 1; A[0][1] = 2; A[0][2] = 3; A[0][3] = 4; // 2nd row A[1][0] = 5; A[1][1] = 6; // 3rd row A[2][0] = 7; A[2][1] = 8; A[2][2] = 9; System.out.println("\nArray Representation"); for(int i = 0; i < A.length; i++) { for(int j = 0; j < A[i].length; j++) { System.out.print(A[i][j] + " "); } System.out.println(" "); } } }
Output
Total Number of Rows: 3
Array Representation
1 2 3 4
5 6
7 8 9
Summary:
In this article, we understood the Different Data Types in Java Program – Java Tutorial. If you like the tutorial share it with your friends. Like the Facebook page for regular updates and the YouTube channel for video tutorials.